As a Swift developer, it’s important to follow coding standards to ensure that your code is clean, easy to read, and maintainable. Following these standards not only makes it easier for other developers to understand your code but also makes it easier for you to understand your own code in the future. In this blog post, we’ll discuss some of the coding standards that Swift developers should take care of before coding.
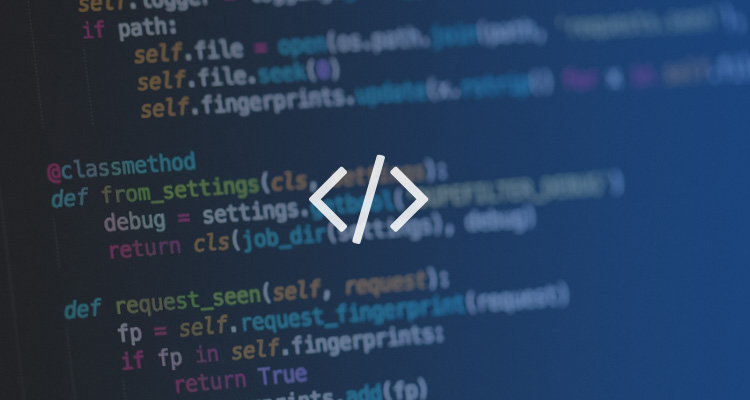
- Use Proper Indentation
Proper indentation is essential for making your code easy to read. Swift uses curly braces {} to define code blocks, and each code block should be indented properly. Here’s an example:
//Swift code
if condition {
// Code block
} else {
// Code block
}
- Use Descriptive Names for Variables and Functions
Using descriptive names for variables and functions is essential for making your code easy to understand. Avoid using abbreviations or single-letter variable names. Instead, use names that accurately describe the purpose of the variable or function. For example:
//swift code
let numberOfItemsInCart: Int = 0
func calculateTotalPrice(for items: [Item]) -> Double {
// Code block
}
- Follow the Swift API Design Guidelines
Apple has provided a set of guidelines for designing Swift APIs, which developers should follow to ensure consistency across the platform. Some of the guidelines include:
- Use camel case for variable and function names.
- Use lowercase letters for the first word of a function name, and uppercase letters for subsequent words.
- Use external parameter names for all arguments in a function.
- Use optionals only when necessary.
- Use type inference whenever possible.
- Use Optionals Carefully
Optionals in Swift allow developers to represent the absence of a value. However, using too many optionals can make the code difficult to read and maintain. Use optionals only when necessary, and provide default values whenever possible. For example:
//swift code
var optionalValue: String? // Optional
var nonOptionalValue: String // Non-optional
var optionalValueWithDefaultValue: String = "default" // Optional with default value
- Use Enums for Grouping Related Values
Swift provides enums, which are a great way to group related values together. Using enums can make the code more readable and maintainable. For example:
//swift code
enum Direction {
case north
case south
case east
case west
}
- Use Structs for Simple Data Types
In Swift, structs are used to define simple data types that are passed around by value. Use structs to represent simple data types, such as coordinates or colors. For example:
//swift code
struct Coordinate {
var latitude: Double
var longitude: Double
}
- Use Comments to Document Your Code
Using comments to document your code can help other developers understand your code and its purpose. Use comments to explain complex or non-obvious code, and to describe the purpose of functions and variables. For example:
//swift code
// This function calculates the area of a rectangle
func calculateArea(length: Double, width: Double) -> Double {
return length * width
}
Following these coding standards will make your code more readable, maintainable, and consistent. It will also make it easier for other developers to work with your code. Happy coding!
I hope you found this blog post helpful. If you have any questions or suggestions, please leave a comment below.
Leave a Reply